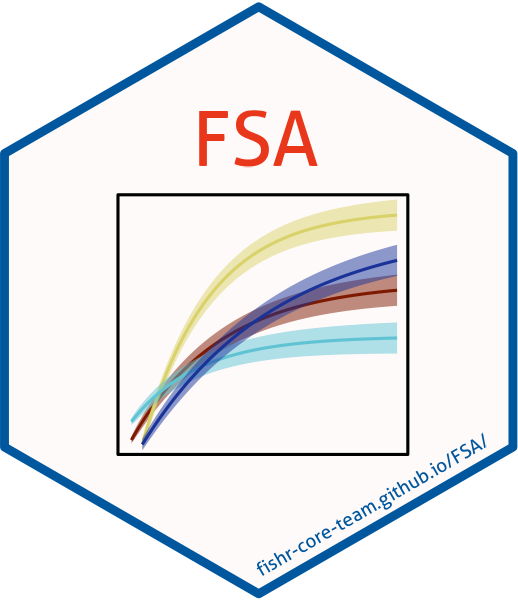
Computes a vector of relative weights specific to a species in an entire data frame.
Source:R/wrAdd.R
wrAdd.Rd
This computes a vector that contains the relative weight specific to each species for all individuals in an entire data frame.
Arguments
- wt
A numeric vector that contains weight measurements or a formula of the form
wt~len+spec
where “wt” generically represents the weight variable, “len” generically represents the length variable, and “spec” generically represents the species variable. Note that this formula can only contain three variables and they must be in the order of weight first, length second, species third.- ...
Not used.
- len
A numeric vector that contains length measurements. Not used if
wt
is a formula.- spec
A character or factor vector that contains the species names. Not used if
wt
is a formula.- units
A string that indicates whether the weight and length data in
formula
are in ("metric"
(DEFAULT; mm and g) or"English"
(in and lbs) units.- data
A data.frame that minimally contains variables of the the observed lengths, observed weights, and the species names given in the
formula=
.
Value
Returns A numeric vector that contains the computed relative weights, in the same order as in data=
.
Details
This computes a vector that contains the relative weight specific to each species for all individuals in an entire data frame. The vector can be appended to an existing data.frame to create a variable that contains the relative weights for each individual. The relative weight value will be NA
for each individual for which a standard weight equation does not exist in WSlit
, a standard weight equation for the units given in units=
does not exist in WSlit
, a standard weight equation for the 75th percentile does not exist in WSlit
, or if the individual is shorter or longer than the lengths for which the standard weight equation should be applied. Either the linear or quadratic equation has been listed as preferred for each species, so only that equation will be used. The use of the 75th percentile is by far the most common and, because this function is designed for use on entire data frames, it will be the only percentile allowed. Thus, to use equations for other percentiles, one will have to use “manual” methods. See WSlit
and wsVal
for more details about types of equations, percentiles, finding which species have published standard weight equations, etc. See the examples for one method for changing species names to something that this function will recognize.
References
Ogle, D.H. 2016. Introductory Fisheries Analyses with R. Chapman & Hall/CRC, Boca Raton, FL.
Author
Derek H. Ogle, DerekOgle51@gmail.com
Examples
## Create random data for three species
# just to control the randomization
set.seed(345234534)
dbt <- data.frame(species=factor(rep(c("Bluefin Tuna"),30)),
tl=round(rnorm(30,1900,300),0))
dbt$wt <- round(4.5e-05*dbt$tl^2.8+rnorm(30,0,6000),1)
dbg <- data.frame(species=factor(rep(c("Bluegill"),30)),
tl=round(rnorm(30,130,50),0))
dbg$wt <- round(4.23e-06*dbg$tl^3.316+rnorm(30,0,10),1)
dlb <- data.frame(species=factor(rep(c("Largemouth Bass"),30)),
tl=round(rnorm(30,350,60),0))
dlb$wt <- round(2.96e-06*dlb$tl^3.273+rnorm(30,0,60),1)
df <- rbind(dbt,dbg,dlb)
str(df)
#> 'data.frame': 90 obs. of 3 variables:
#> $ species: Factor w/ 3 levels "Bluefin Tuna",..: 1 1 1 1 1 1 1 1 1 1 ...
#> $ tl : num 1371 1558 2031 2226 2124 ...
#> $ wt : num 19231 38147 90530 104718 88761 ...
df$Wr1 <- wrAdd(wt~tl+species,data=df)
## same but with non-formula interface
df$Wr2 <- wrAdd(df$wt,df$tl,df$species)
## Same as above but using dplyr
if (require(dplyr)) {
df <- mutate(df,Wr3a=wrAdd(wt,tl,species))
}
df
#> species tl wt Wr1 Wr2 Wr3a
#> 1 Bluefin Tuna 1371 19231.0 NA NA NA
#> 2 Bluefin Tuna 1558 38146.9 NA NA NA
#> 3 Bluefin Tuna 2031 90530.4 NA NA NA
#> 4 Bluefin Tuna 2226 104718.3 NA NA NA
#> 5 Bluefin Tuna 2124 88760.7 NA NA NA
#> 6 Bluefin Tuna 1685 62842.5 NA NA NA
#> 7 Bluefin Tuna 1951 79294.6 NA NA NA
#> 8 Bluefin Tuna 2136 88992.9 NA NA NA
#> 9 Bluefin Tuna 1638 50819.4 NA NA NA
#> 10 Bluefin Tuna 2060 84458.4 NA NA NA
#> 11 Bluefin Tuna 1857 64052.5 NA NA NA
#> 12 Bluefin Tuna 2382 125738.2 NA NA NA
#> 13 Bluefin Tuna 2021 85658.6 NA NA NA
#> 14 Bluefin Tuna 1862 58800.5 NA NA NA
#> 15 Bluefin Tuna 1814 60758.3 NA NA NA
#> 16 Bluefin Tuna 1953 78388.3 NA NA NA
#> 17 Bluefin Tuna 2079 79556.7 NA NA NA
#> 18 Bluefin Tuna 1840 66489.0 NA NA NA
#> 19 Bluefin Tuna 2049 92100.8 NA NA NA
#> 20 Bluefin Tuna 2545 142705.1 NA NA NA
#> 21 Bluefin Tuna 2037 100179.1 NA NA NA
#> 22 Bluefin Tuna 1310 16968.9 NA NA NA
#> 23 Bluefin Tuna 2028 79251.3 NA NA NA
#> 24 Bluefin Tuna 1767 49999.8 NA NA NA
#> 25 Bluefin Tuna 1970 76008.7 NA NA NA
#> 26 Bluefin Tuna 1866 61425.8 NA NA NA
#> 27 Bluefin Tuna 1777 56046.1 NA NA NA
#> 28 Bluefin Tuna 1682 55881.3 NA NA NA
#> 29 Bluefin Tuna 2295 112096.0 NA NA NA
#> 30 Bluefin Tuna 1889 59611.6 NA NA NA
#> 31 Bluegill 158 75.3 91.21118 91.21118 91.21118
#> 32 Bluegill 157 80.3 99.33729 99.33729 99.33729
#> 33 Bluegill 126 26.0 66.70289 66.70289 66.70289
#> 34 Bluegill 203 196.2 103.52426 103.52426 103.52426
#> 35 Bluegill 136 59.9 119.29213 119.29213 119.29213
#> 36 Bluegill 192 159.4 101.17226 101.17226 101.17226
#> 37 Bluegill 132 54.9 120.71165 120.71165 120.71165
#> 38 Bluegill 121 30.7 90.07870 90.07870 90.07870
#> 39 Bluegill 136 42.4 84.44051 84.44051 84.44051
#> 40 Bluegill 52 -5.8 NA NA NA
#> 41 Bluegill 111 17.5 68.35134 68.35134 68.35134
#> 42 Bluegill 226 267.8 98.98901 98.98901 98.98901
#> 43 Bluegill 217 238.2 100.74912 100.74912 100.74912
#> 44 Bluegill 163 86.9 94.93048 94.93048 94.93048
#> 45 Bluegill 119 18.3 56.74628 56.74628 56.74628
#> 46 Bluegill 79 17.9 NA NA NA
#> 47 Bluegill 78 3.5 NA NA NA
#> 48 Bluegill 172 113.9 104.11454 104.11454 104.11454
#> 49 Bluegill 106 28.0 127.42181 127.42181 127.42181
#> 50 Bluegill 122 36.7 104.78446 104.78446 104.78446
#> 51 Bluegill 95 14.1 92.27576 92.27576 92.27576
#> 52 Bluegill 47 -3.3 NA NA NA
#> 53 Bluegill 155 72.8 93.97048 93.97048 93.97048
#> 54 Bluegill 167 101.5 102.31474 102.31474 102.31474
#> 55 Bluegill 146 65.1 102.46768 102.46768 102.46768
#> 56 Bluegill 125 54.5 143.56312 143.56312 143.56312
#> 57 Bluegill 66 -7.1 NA NA NA
#> 58 Bluegill 160 92.1 107.00345 107.00345 107.00345
#> 59 Bluegill 85 1.7 16.08777 16.08777 16.08777
#> 60 Bluegill 72 19.0 NA NA NA
#> 61 Largemouth Bass 351 537.7 84.67395 84.67395 84.67395
#> 62 Largemouth Bass 297 480.0 130.58984 130.58984 130.58984
#> 63 Largemouth Bass 358 598.2 88.30540 88.30540 88.30540
#> 64 Largemouth Bass 451 1435.8 99.53461 99.53461 99.53461
#> 65 Largemouth Bass 375 676.8 85.83334 85.83334 85.83334
#> 66 Largemouth Bass 429 1230.9 100.50555 100.50555 100.50555
#> 67 Largemouth Bass 393 904.5 98.39245 98.39245 98.39245
#> 68 Largemouth Bass 168 104.3 183.16691 183.16691 183.16691
#> 69 Largemouth Bass 358 567.9 83.83256 83.83256 83.83256
#> 70 Largemouth Bass 396 900.0 95.49622 95.49622 95.49622
#> 71 Largemouth Bass 319 481.4 103.65713 103.65713 103.65713
#> 72 Largemouth Bass 325 476.1 96.45038 96.45038 96.45038
#> 73 Largemouth Bass 305 386.9 96.49068 96.49068 96.49068
#> 74 Largemouth Bass 343 505.1 85.77480 85.77480 85.77480
#> 75 Largemouth Bass 359 720.1 105.33403 105.33403 105.33403
#> 76 Largemouth Bass 295 349.4 97.18415 97.18415 97.18415
#> 77 Largemouth Bass 355 561.3 85.17216 85.17216 85.17216
#> 78 Largemouth Bass 336 630.0 114.45429 114.45429 114.45429
#> 79 Largemouth Bass 502 2053.0 100.22722 100.22722 100.22722
#> 80 Largemouth Bass 328 553.9 108.88706 108.88706 108.88706
#> 81 Largemouth Bass 387 899.9 102.94761 102.94761 102.94761
#> 82 Largemouth Bass 324 510.4 104.44721 104.44721 104.44721
#> 83 Largemouth Bass 387 875.1 100.11051 100.11051 100.11051
#> 84 Largemouth Bass 274 243.7 86.31732 86.31732 86.31732
#> 85 Largemouth Bass 437 1347.0 103.53134 103.53134 103.53134
#> 86 Largemouth Bass 313 412.5 94.51644 94.51644 94.51644
#> 87 Largemouth Bass 260 182.4 76.70383 76.70383 76.70383
#> 88 Largemouth Bass 441 1288.2 96.10274 96.10274 96.10274
#> 89 Largemouth Bass 266 265.7 103.69408 103.69408 103.69408
#> 90 Largemouth Bass 371 612.6 80.46670 80.46670 80.46670
## Example with only one species in the data.frame
bg <- droplevels(subset(df,species=="Bluegill"))
bg$Wr4 <- wrAdd(wt~tl+species,data=bg)